Single Layer Perceptron in TensorFlow
TensorFlow 中的单层感知器
In this article, we will be understanding the single-layer perceptron and its implementation in Python using the TensorFlow library. Neural Networks work in the same way that our biological neuron works.
在本文中,我们将了解单层感知器及其在 Python 中使用 TensorFlow 库的实现。神经网络的工作方式与生物神经元的工作方式相同。
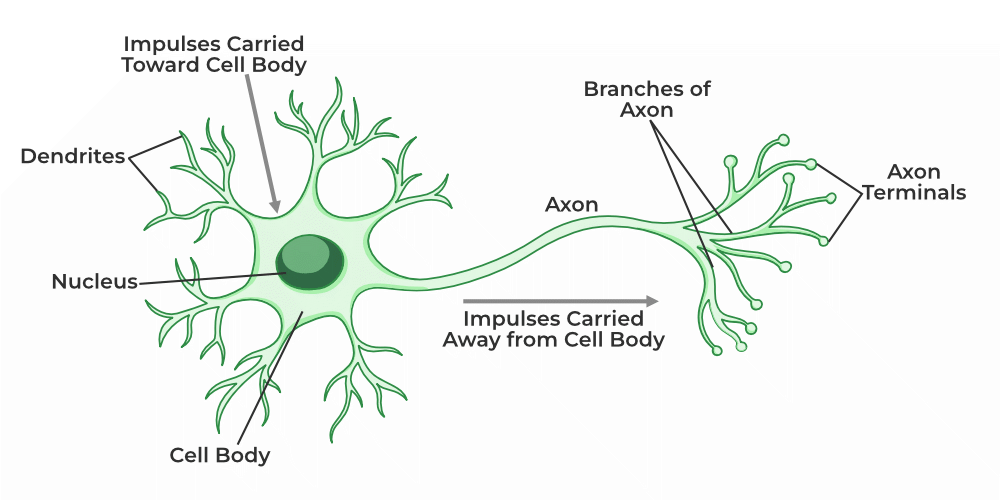
Structure of a biological neuron
生物神经元的结构
Biological neuron has three basic functionality
生物神经元有三种基本功能
- Receive signal from outside.
接收外部信号。 - Process the signal and enhance whether we need to send information or not.
处理信号并增强我们是否需要发送信息。 - Communicate the signal to the target cell which can be another neuron or gland.
将信号传递给目标细胞,目标细胞可以是另一个神经元或腺体。
In the same way, neural networks also work.
神经网络也以同样的方式工作。
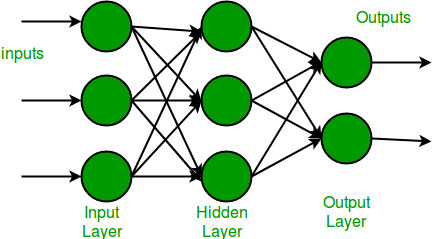
Neural Network in Machine Learning
机器学习中的神经网络
What is Single Layer Perceptron?
什么是单层感知器?
It is one of the oldest and first introduced neural networks. It was proposed by Frank Rosenblatt in 1958. Perceptron is also known as an artificial neural network. Perceptron is mainly used to compute the logical gate like AND, OR, and NOR which has binary input and binary output.
它是最古老、最先引入的神经网络之一。它由弗兰克-罗森布拉特(Frank Rosenblatt)于 1958 年提出。感知器也被称为人工神经网络。感知器主要用于计算二进制输入和二进制输出的逻辑门,如 AND、OR 和 NOR。
The main functionality of the perceptron is:-
感知器的主要功能是
- Takes input from the input layer
从输入层获取输入 - Weight them up and sum it up.
掂量一下它们的重量,然后总结一下。 - Pass the sum to the nonlinear function to produce the output.
将总和传递给非线性函数以产生输出。
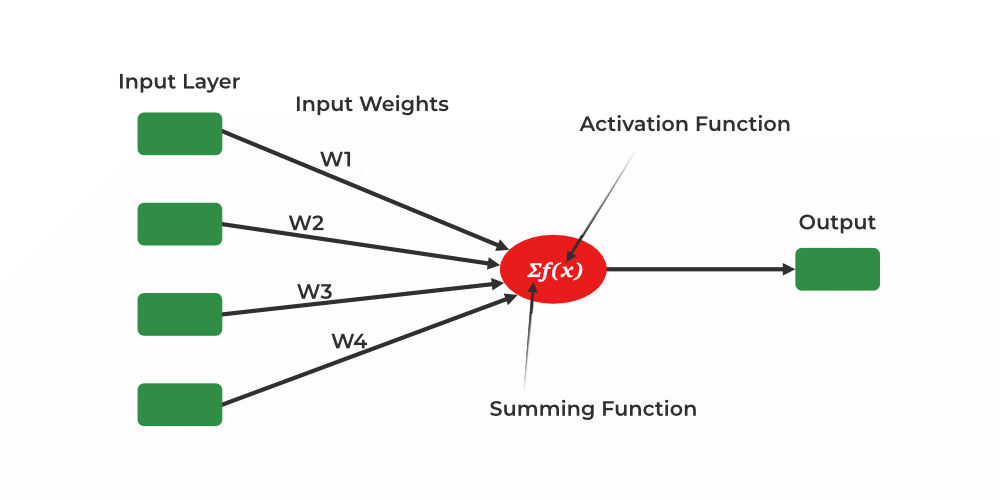
Single-layer neural network
单层神经网络
Here activation functions can be anything like sigmoid, tanh, relu Based on the requirement we will be choosing the most appropriate nonlinear activation function to produce the better result. Now let us implement a single-layer perceptron.
根据要求,我们将选择最合适的非线性激活函数,以产生更好的结果。现在,让我们实现一个单层感知器。
IMPLEMENTATION OF SINGLE-LAYER PERCEPTRON
单层感知器的实现
Let us now implement a single-layer perceptron using the “MNIST” dataset using the TensorFlow library.
现在,让我们使用 TensorFlow 库,利用 "MNIST "数据集实现单层感知器。
Step1: Import necessary libraries
步骤 1:导入必要的库
- Numpy – Numpy arrays are very fast and can perform large computations in a very short time.
Numpy - Numpy 数组速度非常快,可以在很短的时间内完成大量计算。 - Matplotlib – This library is used to draw visualizations.
Matplotlib- 该库用于绘制可视化图像。 - TensorFlow – This is an open-source library that is used for Machine Learning and Artificial intelligence and provides a range of functions to achieve complex functionalities with single lines of code.
TensorFlow - 这是一个用于机器学习和人工智能的开源库,提供一系列功能,只需一行代码即可实现复杂的功能。
- Python3
Python3
import numpy as np import tensorflow as tf from tensorflow import keras import matplotlib.pyplot as plt % matplotlib inline |
Step 2: Now load the dataset using “Keras” from the imported version of tensor flow.
第 2 步:现在使用 "Keras "从导入的张量流版本中加载数据集。
- Python3
Python3
(x_train, y_train),\ (x_test, y_test) = keras.datasets.mnist.load_data() |
Step 3: Now display the shape and image of the single image in the dataset. The image size contains a 28*28 matrix and length of the training set is 60,000 and the testing set is 10,000.
步骤 3:现在显示数据集中单张图像的形状和图像。图像大小包含一个 28*28 的矩阵,训练集的长度为 60,000,测试集的长度为 10,000。
- Python3
Python3
len (x_train) len (x_test) x_train[ 0 ].shape plt.matshow(x_train[ 0 ]) |
Output: 输出:
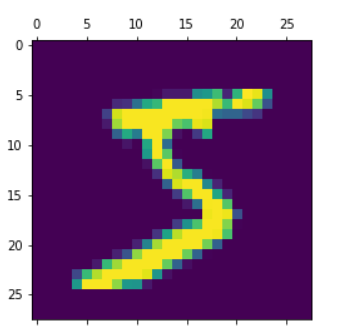
Sample image from the training dataset
训练数据集中的图像样本
Step 4: Now normalize the dataset in order to compute the calculations in a fast and accurate manner.
步骤 4:现在对数据集进行归一化处理,以便快速准确地进行计算。
- Python3
Python3
# Normalizing the dataset x_train = x_train / 255 x_test = x_test / 255 # Flatting the dataset in order # to compute for model building x_train_flatten = x_train.reshape( len (x_train), 28 * 28 ) x_test_flatten = x_test.reshape( len (x_test), 28 * 28 ) |
Step 5: Building a neural network with single-layer perception. Here we can observe as the model is a single-layer perceptron that only contains one input layer and one output layer there is no presence of the hidden layers.
步骤 5:构建单层感知神经网络。这里我们可以看到,由于模型是单层感知器,只包含一个输入层和一个输出层,因此不存在隐藏层。
- Python3
Python3
model = keras.Sequential([ keras.layers.Dense( 10 , input_shape = ( 784 ,), activation = 'sigmoid' ) ]) model. compile ( optimizer = 'adam' , loss = 'sparse_categorical_crossentropy' , metrics = [ 'accuracy' ]) model.fit(x_train_flatten, y_train, epochs = 5 ) |
Output: 输出:
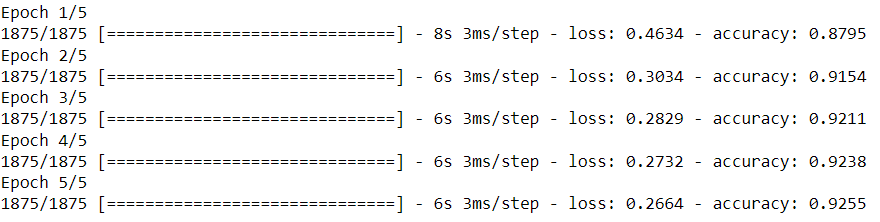
Training progress per epoch
每个纪元的训练进度
Step 6: Output the accuracy of the model on the testing data.
步骤 6:在测试数据上输出模型的准确性。
- Python3
Python3
model.evaluate(x_test_flatten, y_test) |
Output: 输出:

Models performance on the testing data
测试数据的模型性能
Don't miss your chance to ride the wave of the data revolution! Every industry is scaling new heights by tapping into the power of data. Sharpen your skills and become a part of the hottest trend in the 21st century.
千万不要错过在数据革命浪潮中乘风破浪的机会!每个行业都在利用数据的力量攀登新的高峰。磨练你的技能,成为 21 世纪最热门趋势的一部分。
Dive into the future of technology - explore the Complete Machine Learning and Data Science Program by GeeksforGeeks and stay ahead of the curve.
潜入技术的未来--探索 GeeksforGeeks 的完整机器学习和数据科学课程,保持领先地位。
在评论中分享您的想法